Java
Beginners
On Demand
Online
- Java | W3schools https://www.w3schools.com/java/
- Java Tutorial | Javatpoint https://www.javatpoint.com/java-tutorial
- Learn Java | Codecademy https://www.codecademy.com/learn/learn-java
- Java Tutorial For Beginners | Beginnersbook https://beginnersbook.com/java-tutorial-for-beginners-with-examples/
CLASSROOM
- Java Training | Knowledgehunt https://www.knowledgehut.com/programming/java-training
- Java Script | Dotnettricks https://www.dotnettricks.com/learn/javascript
- Core Java Online Training | Onlineitguru https://onlineitguru.com/core-java-online-training-placement.html
- Core Java Training | Gangboard https://www.gangboard.com/app-programming-scripting-training/core-java-training
- Basics Of Java
- Java - What, Where, and Why?
- History and Features of Java
- Internals of Java Program
- Difference between JDK, JRE, and JVM
- Internal Details of JVM
- Variable and Data Type
- Unicode System
- Naming Convention
- OOPS Concepts
- Advantage of OOPs
- Object and Class
- Method Overloading
- Constructor
- Static variable, Method, and Block this keyword
- Inheritance (IS-A)
- Aggregation and Composition(HAS-A)
- Method Overriding
- Covariant Return Type
- Super Keyword
- Instance Initializer block
- final keyword
- Runtime Polymorphism
- static and Dynamic binding
- Abstract class and Interface
- Downcasting with the instance of operator
- Package and Access Modifiers
- Encapsulation
- Object class
- Object Cloning
- Java Array
- Call By Value and Call By Reference
- strictfp keyword
- Creating API Document
- String Handling
- String: What and Why?
- Immutable String
- String Comparison
- String Concatenation
- Substring
- Methods of String class
- StringBuffer class
- StringBuilder class
- Creating the Immutable class
- tostring method
- StringTokenizer class
- Exception Handling
- Exception Handling: What and Why?
- try and catch block
- Multiple catch block
- Nested try
- finally block
- throw keyword
- Exception Propagation
- throws keyword
- Exception Handling with Method Overriding
- Custom Exception
- Nested Classes
- Nested Class: What and Why?
- Member Inner class
- Anonymous Inner class
- Local Inner class
- static nested class
- Nested Interface
- Multithreading
- Multithreading: What and Why?
- Life Cycle of a Thread
- Creating Thread
- Thread Scheduler
- Sleeping a thread
- Joining a thread
- Thread Priority
- Daemon Thread
- Thread Pooling
- Thread Group
- ShutdownHook
- Performing multiple tasks by multiple threads
- Garbage Collection
- Runnable class
- Synchronization
- Synchronization: What and Why?
- synchronized method
- synchronized block
- static synchronization
- Deadlock
- Inter-thread Communication
- Interrupting Thread
- Input & Output
- FileOutputStream & FileInputStream
- ByteArrayOutputStream
- SequenceInputStream
- BufferedOutputStream & BufferedInputStream
- FileWriter & FileReader
- CharArrayWriter
- Input from keyboard by InputStreamReader
- Input from keyboard by Console
- Input from keyboard by Scanner
- PrintStream class
- PrintWriter class
- Compressing and Uncompressing File
- Reading and Writing data simultaneously
- DataInputStream and DataOutputStream
- StreamTokenizer class
- Serialization
- Serialization & Deserialization
- Serialization with IS-A and Has-A
- transient keyword
- Networking
- Socket Programming
- URL class
- Displaying data of a web page
- InetAddress class
- DatagramSocket and DatagramPacket
- Two-way communication
- AWT & EventHandling
- AWT Controls
- Event Handling by 3 ways
- Event classes and Listener Interfaces
- Adapter classes
- Creating Games and Applications
- Swing
- Basics of Swing
- JButton class
- JRadioButton class
- JTextArea class
- JComboBox class
- JTable class
- JColorChooser class
- JProgressBar class
- JSlider class
- Digital Watch
- Graphics in swing
- Displaying Image
- Edit Menu for Notepad
- Open Dialog Box
- Creating Notepad
- Creating Games and applications
- Layout Managers
- BorderLayout
- GridLayout
- FlowLayout
- BoxLayout
- CardLayout
- Applet
- Life Cycle of Applet
- Graphics in Applet
- Displaying image in Applet
- Animation in Applet
- EventHandling in Applet
- JApplet class
- Painting in Applet
- Digital Clock in Applet
- Analog Clock in Applet
- Parameter in Applet
- Applet Communication
- Creating Games
- Reflection API
- Reflection API
- newInstance() & Determining the class object
- javap tool
- creating javap tool
- creating applet viewer
- Accessing private method from outside the class
- Collection
- Collection Framework
- ArrayList class
- LinkedList class
- ListIterator interface
- HashSet class
- LinkedHashSet class
- TreeSet class
- PriorityQueue class
- ArrayDeque class
- Map interface
- HashMap class
- LinkedHashMap class
- TreeMap class
- Hashtable class
- Comparable and Comparator
- Properties class
- JDBC
- JDBC Drivers
- Steps to connect to the database
- Connectivity with Oracle
- Connectivity with MySQL
- Connectivity with Access without DSN
- DriverManager
- Connection interface
- Statement interface
- ResultSet interface
- PreparedStatement
- ResultSetMetaData
- DatabaseMetaData
- Storing image
- Retrieving image
- Storing file
- Retrieving file
- Stored procedures and functions
- Transaction Management
- Batch Processing
- JDBC New Features
- Mini Project
- Java New Features
- Assertion
- For-each loop
- Varargs
- Static Import
- Autoboxing and Unboxing
- Enum Type
- Annotation etc.
- Internationalization
- Internationalization
- ResourceBundle class
- I18N with Date
- I18N with Time
- I18N with Number
- I18N with Currency
Advanced
On Demand
Online
- Jsp Tutorial | Javatpoint https://www.javatpoint.com/jsp-tutorial
- Advanced Java Tutorials | Roseindia https://www.roseindia.net/java/Advanced-Java-Tutorials.shtml
- Advanced Java | Javacodegeeks https://www.javacodegeeks.com/2015/09/advanced-java.html
CLASSROOM
- Advanced Java Online Training | Naresh IT https://nareshit.com/advanced-java-online-training/
- Java Advanced | Certification Guru https://certificationguru.co.in/javaadvanced.html
- Advanced Java | Edureka https://www.edureka.co/advanced-java-sp
- Java Development Training | Simplilearn https://www.simplilearn.com/mobile-and-software-development/java-javaee-soa-development-training
Servlet
- Basics Of Servlet
- Servlet: What and Why?
- Basics of Web
- Servlet API
- Servlet Interface
- GenericServlet
- HttpServlet
- Servlet Life Cycle
- Working with Apache Tomcat Server
- Steps to create a servlet in Tomcat
- How servlet works?
- servlet in Myeclipse
- servlet in Eclipse
- servlet in Netbeans
- ServletRequest
- ServletRequest methods
- Registration example with DB
- Servlet Collaboration
- RequestDispatcher
- sendRedirect
- ServletConfig
- ServletConfig methods
- ServletConfig example
- ServletContext
- ServletContext methods
- ServletContext example
- Attribute
- How to set, get, and remove example?
- Session Tracking
- Cookies
- Hidden Form Field
- URL Rewriting
- HttpSession
- Event & Listener
- Filter
- Filter
- Authentication Filter
- FilterConfig
- Useful examples
- ServletInputStream and ServletOutputStream
- Annotation Servlet
- Project Development
JSP
- Basics Of JSP
- Life cycle of JSP
- JSP API
- JSP in Eclipse and other IDE's
- Scripting Elements
- scriptlet tag
- expression tag
- declaration tag
- Implicit Objects
- out
- request
- response
- config
- application
- session
- pageContext
- page
- exception
- Directive Elements
- page directive
- include directive
- taglib directive
- Exception Handling
- Action Elements
- JSP:forward
- JSP:include
- Bean class
- JSP:useBean
- JSP:setProperty & JSP:getProperty
- Displaying applet in JSP
- Expressing Language
- MVC in JSP
- JSTL
- Custom Tags
- Custom Tag: What and Why?
- Custom Tag API?
- Custom Tag Example
- Attributes
- Iteration
- Custom URI
- Project Development in JSP
JavaMail API
- Sending Email
- Sending email through Gmail server
- Receiving Email
- Sending HTML content
Design Pattern
- Singleton
- DAO
- DTO
- MVC
- Front Controller
- Factory Method etc..
JUnit
- JUnit: What and Why?
- Types of Testing
- Annotations used in JUnit
- Assert class
- Test Cases
Maven
- Maven: What and Why?
- Ant Vs Maven
- How to install Maven?
- Maven Repository
- Understanding pom.xml
- Maven Example
- Maven Web App Example
- Maven using Eclipse
Struts 2
- Basics of Struts2
- Struts: What and Why?
- Model1 vs Model2
- Struts2 Features
- Steps to create Struts2 application
- Understanding Action class
- Understanding struts.xml file
- Struts2 in Eclipse IDE
- Struts2 in Myeclipse IDE
- Core Components
- Interceptors
- ValueStack
- ActionContext
- ActionInvocation
- OGNL
- Struts2 Architecture
- Struts2 Action
- Action Interface
- ActionSupport class
- Struts2 Config
- multi-configuration
- multi namespace
- Interceptors
- Custom Interceptor: Life Cycle of Interceptor
- params interceptor
- execAndWait interceptor
- prepare interceptor
- modelDriven interceptor
- exception interceptor
- fileUpload interceptor
- Stuts2 Validation
- Custom Validation: workflow interceptor
- Input Validation: validation interceptor
- Ajax Validation: jsonValidation interceptor
- Aware Interfaces
- ServletActionContext
- SessionAware
- Login and Logout Application
- ServletContextAware
- Struts2 with I18N
- Zero Configuration
- By convention
- By annotation
- Struts2 with Tiles2
- Hibernate with Struts2
- Spring With Struts2
- Project Development in Struts2
Working With IDE
- Eclipse IDE
- Netbeans IDE
- Myeclipse IDE
Working with Servers
- Apache Tomcat
- Glassfish Server
- JBoss Server
- Weblogic Server
EJB3
- Introduction To JavaEE
- The Need for JavaEE
- Overview of the JavaEE Architecture
- 1 tier
- 2 tier
- 3 tier
- N tier
- JavaEE Key Standards
- Introduction to EJB3
- The EJB Model
- Key Services of the Application Server
- Developing Session Beans
- Stateless Session Beans
- Stateful Session Beans
- Packaging
- Writing Clients
- Using Dependency Injection
- No More JNDI
- Injection of EJBContext
- JMS
- JMS Overview
- JMS Messaging Domains
- Example of JMS using Queue
- Example of JMS using Topic
- Message Driven Beans
- Persistence Introduction to JPA
- Object Relational Mapping
- Mapping configurations
- Embedded Objects
- EJB QL
Web Technology
- HTML5
- Introduction to HTML
- HTML Tags
- Creating Forms
- Creating tables
- Managing home page
- CSS
- Introduction to CSS
- Three ways to use CSS
- CSS Properties
- Designing website
- Working with Templates
- Javascript
- Introduction to Javascript
- Three ways to use Javascript
- Working with events
- Client-side Validation
- JQuery
- Introduction to JQuery
- Validation using JQuery
- JQuery Forms
- JQuery Examples
- AJAX
- Introduction to AJAX
- Servlet and JSP with AJAX
- Interacting with database
Certifications
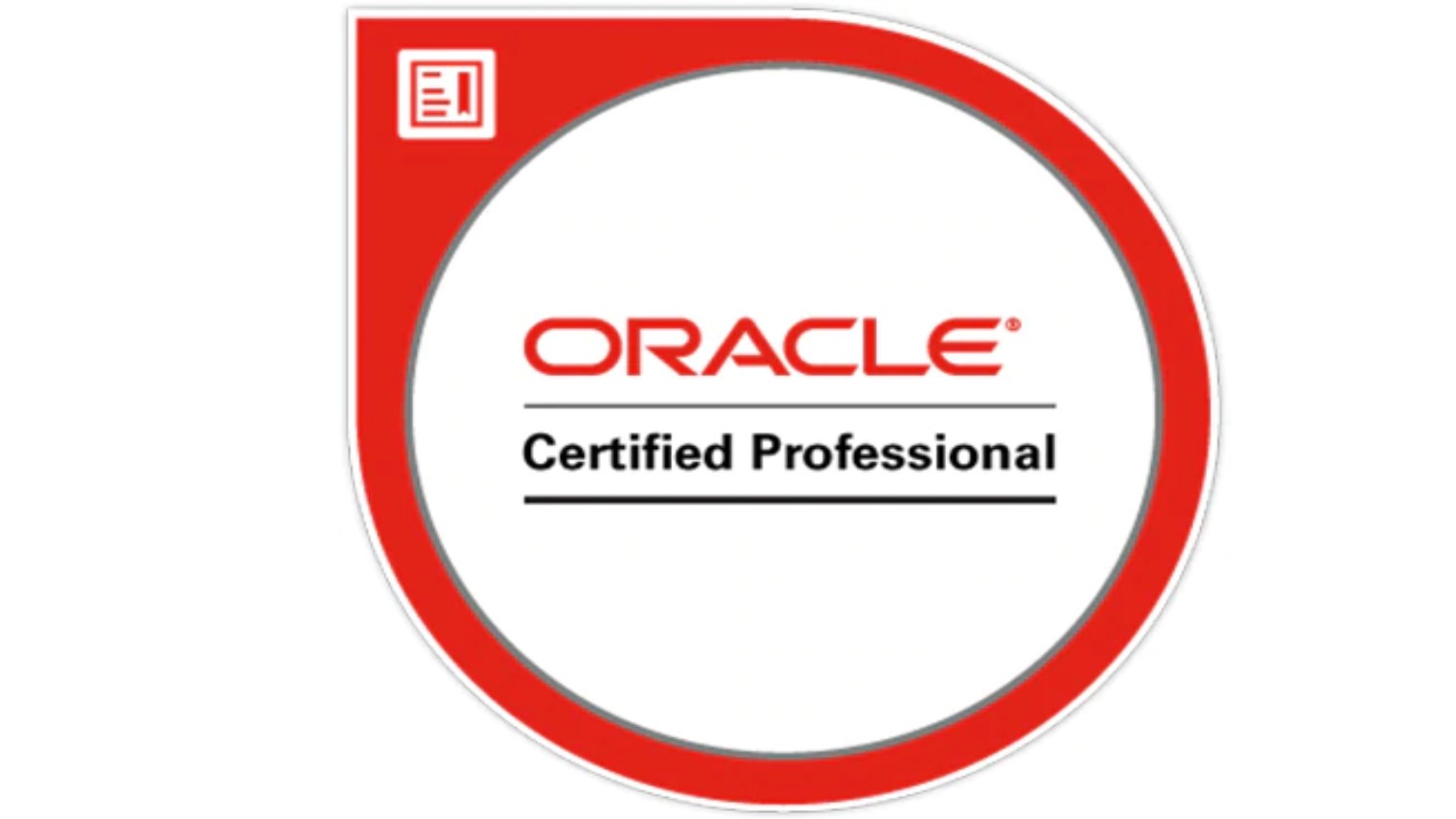
Oracle Certified Professional: Java SE 11 Developer | Education oracle
This course includes- - Java SE 11 Developer, you will demonstrate proficiency in Java, a thorough and broad knowledge of Java programming language
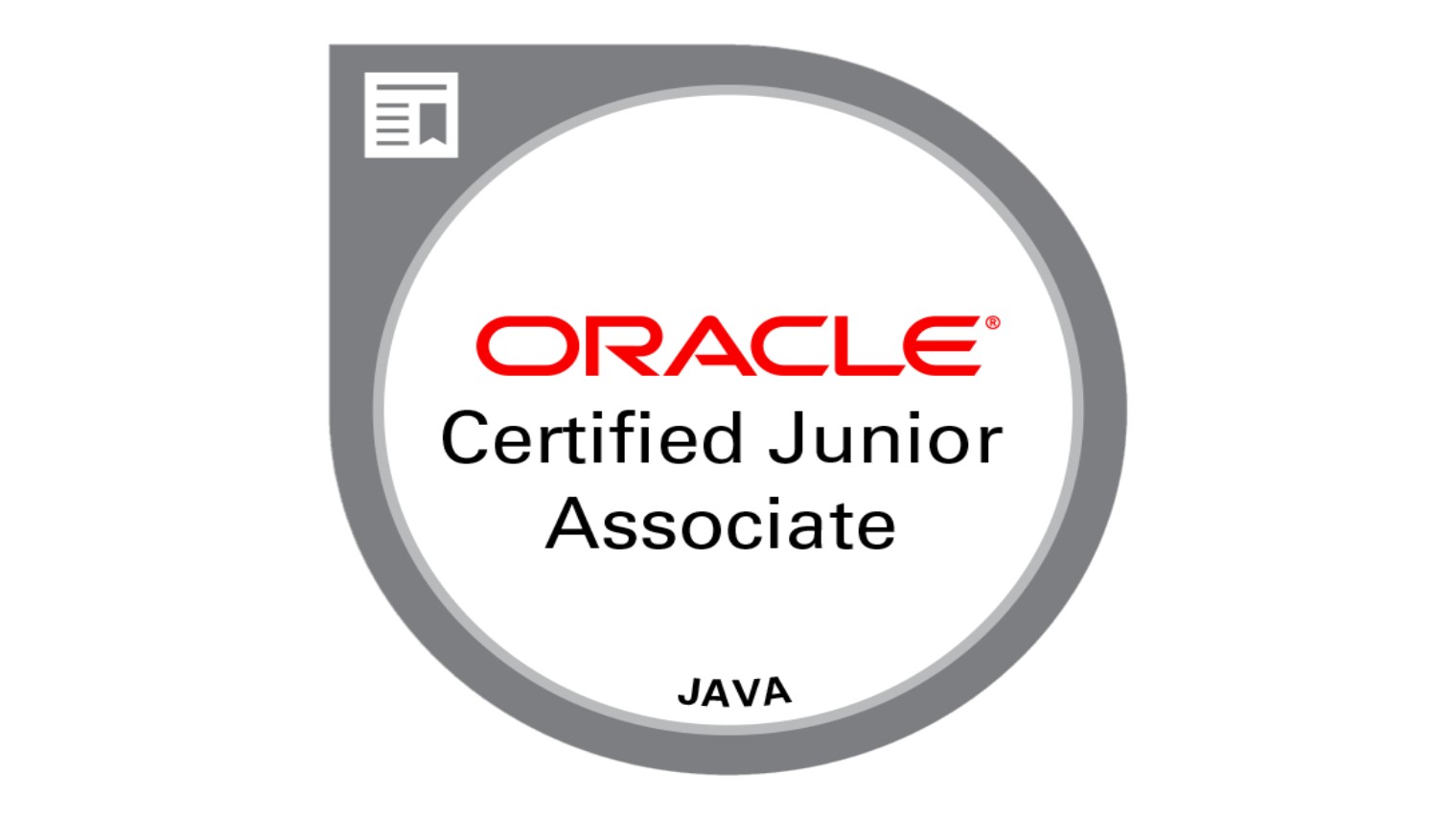
Java Foundations Certified Junior Associate | education oracle
This course includes- - This Junior Associate certification is focused on students in secondary schools, two-year colleges and four year colleges and universities who have participated in the Oracle Academy program and/or are studying computer science including relevant Java curricula and faculty members who teach foundational Java and computer science classes.